Microsoft just recently put into preview a new feature called Web Tiles for getting external data onto your Band. Previously to get data from an external source onto your Band you needed to write a custom mobile app using the Band SDK to create the tile, pull in the data, and push to the device. With a Web Tile you can read data from JSON or XML web services (including ATOM & RSS feeds) and pick how it will lay out on your device using the drop dead simple tile designer.
Obviously there are things to consider before deciding to use a Web Tile or create a mobile app. With a Web Tile the service must not require any type of authentication, so whatever data you are making available shouldn’t be sensitive (security by obscurity I guess). By default the Microsoft Health app that backs the Band will only refresh the data every 30 minutes (could be lowered to 15 based on the documentation). There is no alerting mechanism, so you won’t get notifications when data changes and at any given point the data could be outdated. On the plus side for casual data consumption deploying a web service that returns JSON and creating some Web Tiles is probably going to be way easier than writing a mobile application (especially if you are supporting multiple devices).
As a sample I created a simple web service using Web API that lives on Azure to provide data. In this case I’m reading from CRM and providing a count of new Opportunity records created for a user for the day. Again since there no authentication in place – don’t expose sensitive data!
The service accepts the system user id of the individual whom we need the data for. It returns that user’s full name, a message describing the output, and the number of Opportunity records created for the day - ultimately all the data we are going to show on the Band.
Here’s what the web service code looks like:
On a side note if you aren’t already familiar with Swagger for documenting and testing RESTful APIs it would be worth checking out. Specifically for Web API you can install Swashbuckle from NuGet which at the very least gives you a slick UI for testing.
Getting the data ready and in a useable format was the hard part. Creating the Web Tile is very easy, it’s all done through the tile designer website.
Here’s what that process looks like.
We have a few different layout options to choose from.
![1s 1s]()
Next provide the url to your web service. In this case I’m providing the specific user id for the user whom I need the data for in the query string. Obviously this means you need to configure a tile for each user consuming this service as there isn’t currently a way to get this data dynamically.
![2s 2s]()
Then simply drag fields from your result to the corresponding locations on the tile.
![3s 3s]()
Next provide some information about the tile and upload the image that will be displayed on the Band – I hijacked the CRM Opportunity icon.
![4s 4s]()
Finally you’ll be able to download the web tile file. You’ll need to open it on your mobile device with Microsoft Health installed so either email it to yourself or open it from OneDrive. From there you’ll be prompted to create the tile on your Band. You’ll see the square image below in the list of tiles and selecting it will show the data being pulled from the web service based on the layout you’ve selected.
![5s 5s]()
And that’s it. The data should be periodically refreshed without any further intervention.
You can grab the code here:
https://github.com/jlattimer/CRMWebTileExample
Obviously there are things to consider before deciding to use a Web Tile or create a mobile app. With a Web Tile the service must not require any type of authentication, so whatever data you are making available shouldn’t be sensitive (security by obscurity I guess). By default the Microsoft Health app that backs the Band will only refresh the data every 30 minutes (could be lowered to 15 based on the documentation). There is no alerting mechanism, so you won’t get notifications when data changes and at any given point the data could be outdated. On the plus side for casual data consumption deploying a web service that returns JSON and creating some Web Tiles is probably going to be way easier than writing a mobile application (especially if you are supporting multiple devices).
As a sample I created a simple web service using Web API that lives on Azure to provide data. In this case I’m reading from CRM and providing a count of new Opportunity records created for a user for the day. Again since there no authentication in place – don’t expose sensitive data!
The service accepts the system user id of the individual whom we need the data for. It returns that user’s full name, a message describing the output, and the number of Opportunity records created for the day - ultimately all the data we are going to show on the Band.
Here’s what the web service code looks like:
[HttpGet]
public JsonResult<NewOppCount> Get(string userId)
{
OrganizationService orgService;
CrmConnection connection = CrmConnection.Parse(
ConfigurationManager.ConnectionStrings["CRMConnectionString"].ConnectionString);
using (orgService = new OrganizationService(connection))
{
//Query the CRM data based on the user id being passed in
FetchExpression query = new FetchExpression(@"
<fetch distinct='true' aggregate='true' >
<entity name='opportunity' >
<attribute name='opportunityid' alias='NewOppCount' aggregate='count' />
<attribute name='ownerid' alias='ownerid' groupby='true' />
<filter type='and' >
<condition attribute='ownerid' operator='eq' value='" + userId + @"' />
<condition attribute='createdon' operator='today' />
</filter>
</entity>
</fetch>");
//Get the result values for output
EntityCollection results = orgService.RetrieveMultiple(query);
string username =
(string)results.Entities[0].GetAttributeValue<AliasedValue>("ownerid_owneridname").Value;
int count =
(int)results.Entities[0].GetAttributeValue<AliasedValue>("NewOppCount").Value;
NewOppCount result = new NewOppCount
{
Username = username,
Message = "New Opps Today:",
Count = count
};
//Return JSON or XML
return Json(result);
}
}
On a side note if you aren’t already familiar with Swagger for documenting and testing RESTful APIs it would be worth checking out. Specifically for Web API you can install Swashbuckle from NuGet which at the very least gives you a slick UI for testing.
Getting the data ready and in a useable format was the hard part. Creating the Web Tile is very easy, it’s all done through the tile designer website.
Here’s what that process looks like.
We have a few different layout options to choose from.
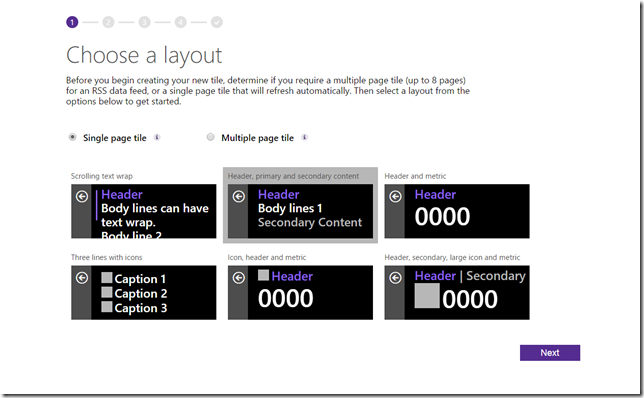
Next provide the url to your web service. In this case I’m providing the specific user id for the user whom I need the data for in the query string. Obviously this means you need to configure a tile for each user consuming this service as there isn’t currently a way to get this data dynamically.
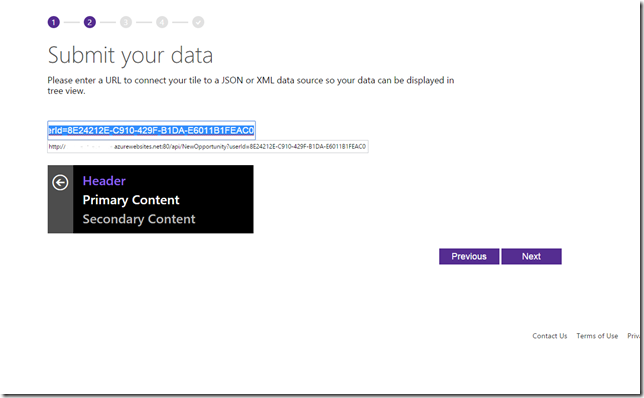
Then simply drag fields from your result to the corresponding locations on the tile.
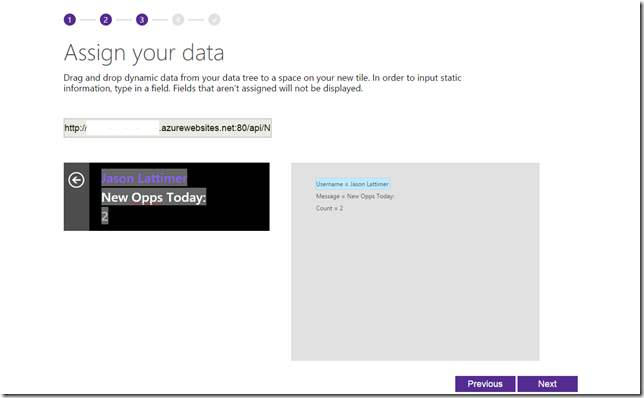
Next provide some information about the tile and upload the image that will be displayed on the Band – I hijacked the CRM Opportunity icon.
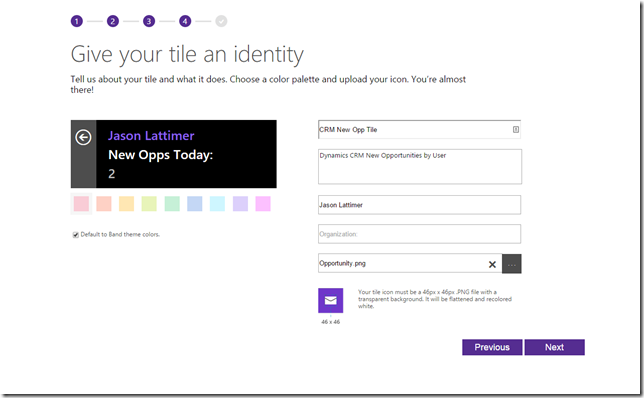
Finally you’ll be able to download the web tile file. You’ll need to open it on your mobile device with Microsoft Health installed so either email it to yourself or open it from OneDrive. From there you’ll be prompted to create the tile on your Band. You’ll see the square image below in the list of tiles and selecting it will show the data being pulled from the web service based on the layout you’ve selected.
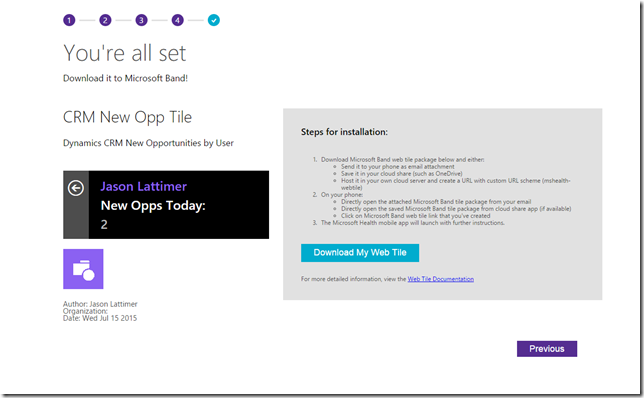
And that’s it. The data should be periodically refreshed without any further intervention.
You can grab the code here:
https://github.com/jlattimer/CRMWebTileExample